开发 Saber 项目使用 VSCode 作为编辑器。Saber 项目使用 @vue/cli 创建的,在此基础上再进行代码检查相关配置,包括拼写检查、JavaScript 代码检查、CSS 代码检查、代码风格检查、使用 Git Hook 工具进行提交前代码规范校验。
拼写检查
- VSCode 中添加 Code Spell Checker 插件,进行单词的拼写检查,如果代码中出现了拼写错误编辑器会有提示
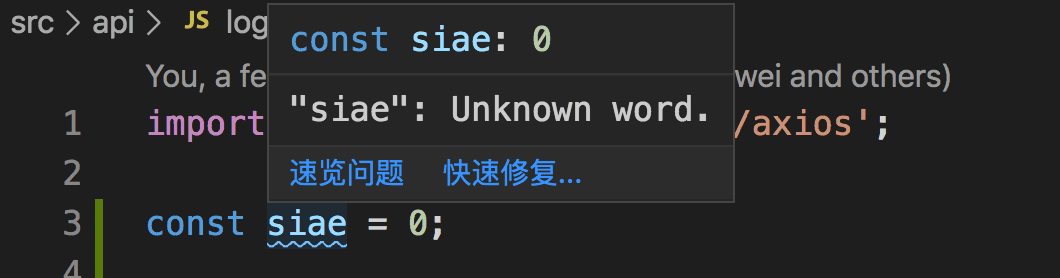
JS 检查
- VSCode 中添加 ESLint 插件,参考 ESLint 官网 进行配置,进行 JavaScript 语法和格式检查
- 项目安装 eslint-plugin-import、eslint-plugin-node、eslint-plugin-promise、eslint-plugin-vue 包
- 项目根目录添加 .eslintrc.js 文件,内容如下
|
|
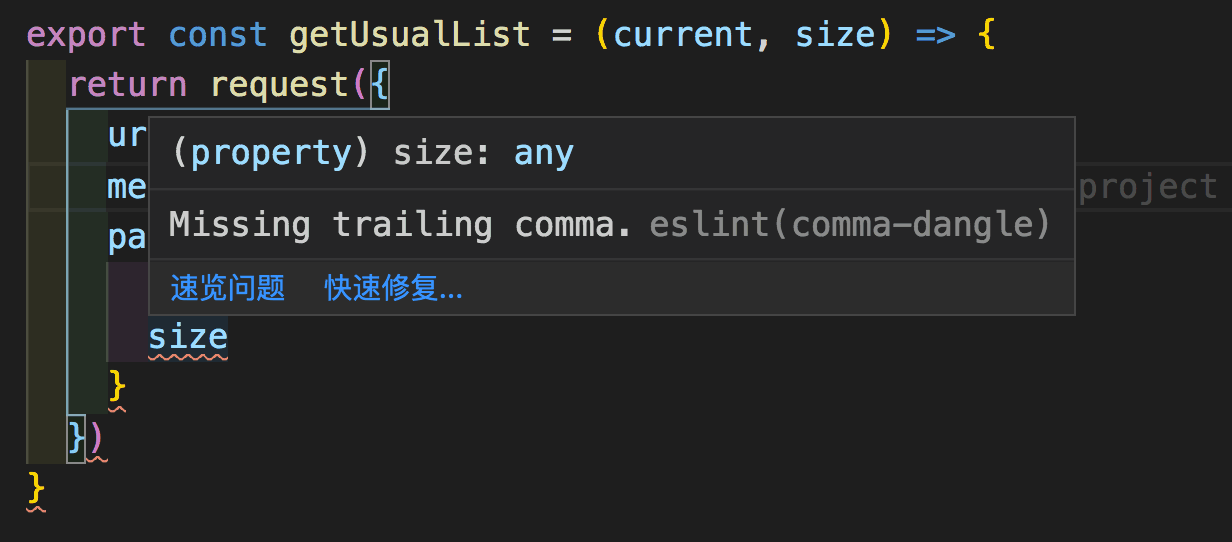
CSS 检查
- VSCode 中添加 stylelint 插件,参考 stylelint 进行配置,进行 CSS 语法和格式检查
- 项目添加 stylelint、stylelint-config-standard、stylelint-config-prettier、stylelint-order 包
- 项目根目录添加 .stylelintrc.js 文件,内容如下
|
|
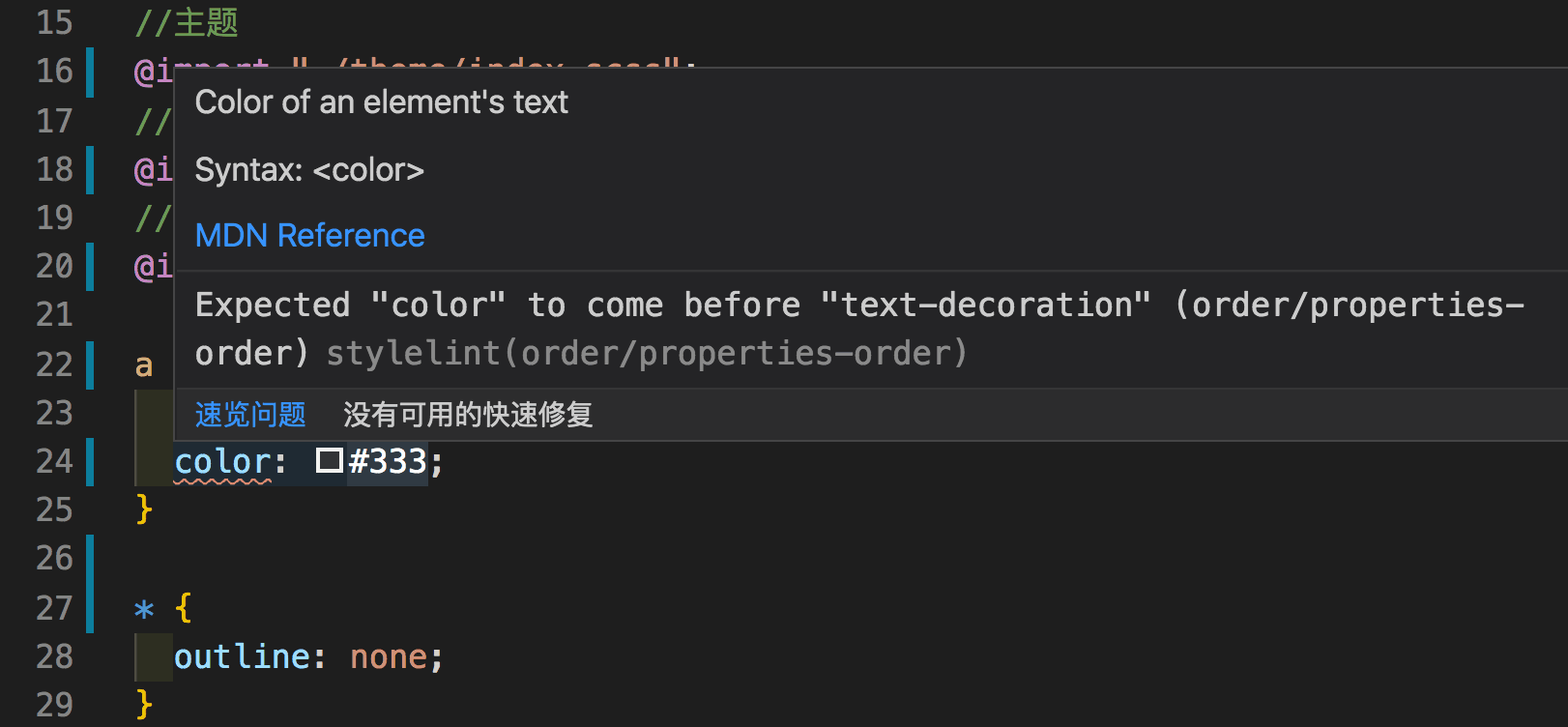
格式检查
- VSCode 中添加 Prettier - Code formatter 插件,参考 prettier 官网 进行配置,配置完成可用 Alt + Shift + F 进行代码格式化
- 项目添加 eslint-config-prettier、eslint-plugin-prettier 包
- 项目根目录添加 .prettierrc.js 文件,内容如下
|
|
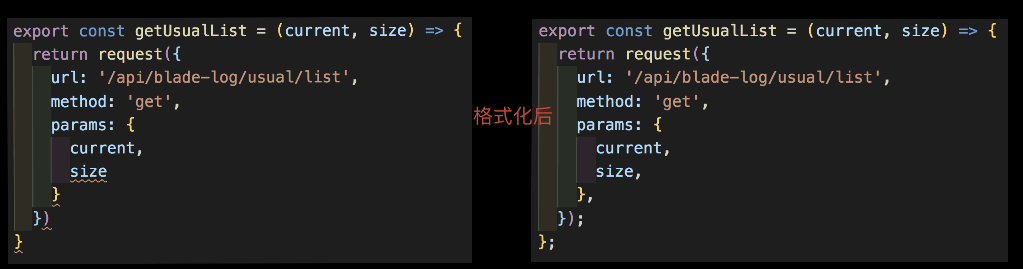
Git Hook
- 利用 git 的 hooks 机制,在提交 commit 时自动调用格式校验
- 项目安装 husky、lint-staged 包
- package.json 中添加如下内容
|
|
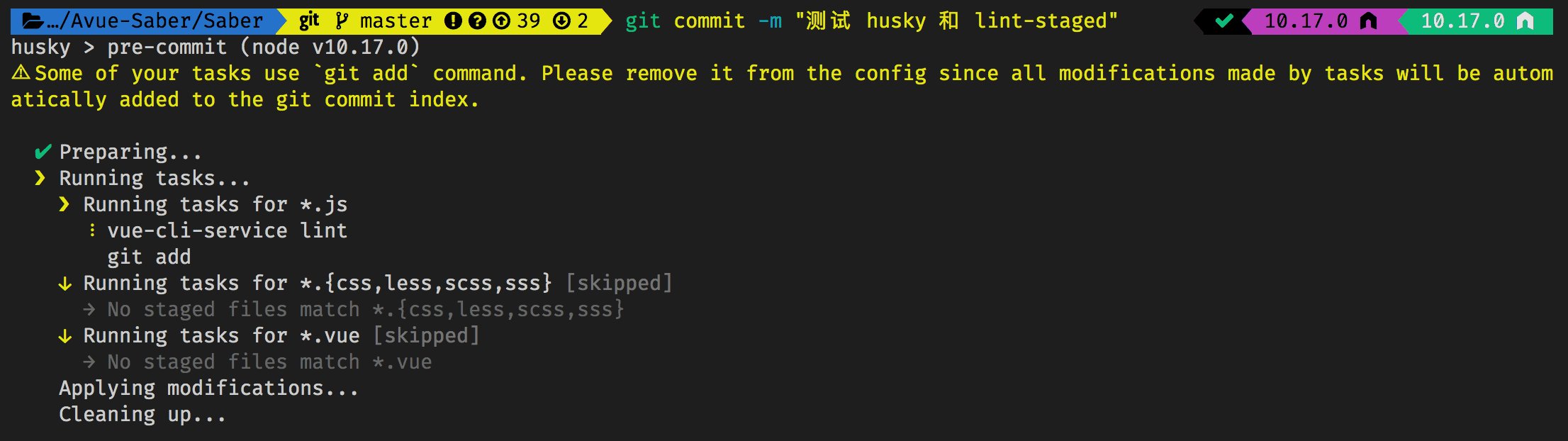